Lately I have been working on several web applications, both as hobby projects and at work. I started using YUI3 a few years ago as a Javascript framework, and I liked it. But I kept hearing about jQuery, and the times I saw code snippets, I was intrigued. It looked different, but at the same time jQuery seemed very powerful and efficient.
So a while back I started looking closer at jQuery, and I found that it was extremely easy to learn. One need a decent understanding of HTML and the browser DOM (Document Object Model), as well as Javascript knowledge. Add some CSS to that, if you want the page to look good as well, and you are set.
So how do you start using jQuery? The easy way is to take advantage of companies like Google and Microsoft who are hosting different frameworks (including jQuery) on their servers for public use. You don’t have to worry about downloading and hosting it yourself, and you can get started in just minutes.
You add code to your page to utilize jQuery, then add some script. You have to allow the browser to wait for the webpage to fully load before you can start doing things, and this is done using $(document).ready(). When that event is triggered, the code you have added there will be executed.
It is very easy to address elements on your webpage. If I have an element (could be a button, a span/div section, a link or even an image) with the id “messageBox”, I can address it like this: $(“#messageBox”). I then have different properties and methods for that element.
But I have always believed in “show me the code”. I created a very simple demo. The webpage contains a button and a div where we want to display a text when the button is clicked. I have some CSS to make the text look nice, and a few lines of jQuery code to do the work.
<html> <head> <title>Hello, jQuery</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.1/jquery.min.js"></script> </head> <script> // The following function is executed after page is fully loaded $(document).ready(function () { // Setup the element with id "btnSave" to react on click $("#btnSave").click( function() { // When clicked, set the innerHTML of the element with // id "messageBox" to the specified html string. $("#messageBox").html("You clicked the <strong>Save</strong> button."); $("#messageBox").addClass("statusMessage"); }); }); </script> <style> .statusMessage { font-family: Arial; font-size: 0.9em; color: #AA0000; } </style> <body> <button id="btnSave">Save</button> <div id="messageBox"></div> </body> </html>
That’s it. Try it yourself, paste this code into a text file and call it jQuery.html, then open it in your browser.
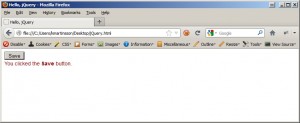
Now when you understand the basics, you can learn more advanced things. I am using jQuery to very easily perform Ajax calls, even calling Lotusscript web agents to return data from a Domino database to my webpage. I also use it for all kinds of dynamic updates to webpages.
A while back I started on a Domino-based web chat as a hobby project. I started building it using YUI3, but after finishing the initial landing page, I started using jQuery on the actual room pages. The other night I simply ripped out the YUI3 code and replaced it with (much less) jQuery code. It took my just an hour or two. The site/chat is currently in Swedish, but I plan to translate it to other languages, and I will blog more about this project soon. Feel free to try it out, or just look at the source code for the pages. I am sure you will very quickly understand how it works. On that page I use jQuery to call server agent to return the number of users (and their names) present in the different rooms. I also check values in field, as well as update fields, hiding/showing elements on the page depending on field values, etc.
I also use a jQuery plugin to display bubbles with information (pulled in real time from the Domino database using a web agent) when hovering over the count of users in a given room.